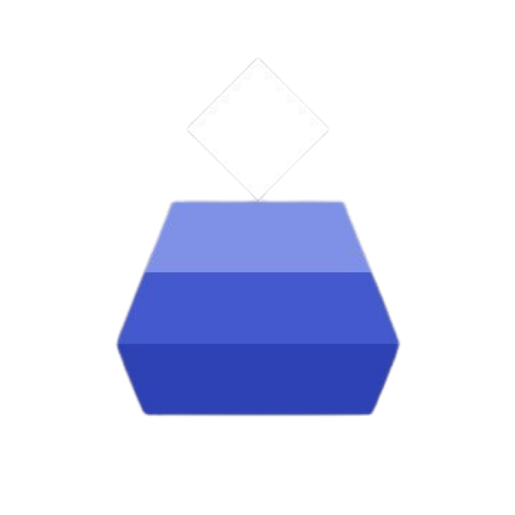
Web3 intelligence at your fingertips
Powerful AI assistance for blockchain development, MEV logic, oracle integration, and more.
Specialized for Web3 development
Advanced Coding
From smart contracts to frontend development, get intelligent code assistance tailored to your needs.
MEV & Data Extraction
Optimize transaction sequencing and extract valuable data from blockchains with expert guidance.
Oracle Integration
Connect your dApps to reliable data sources with real-time oracle implementation support.
And much, much more...
Experience BaseAI in action
See how BaseAI can help accelerate your Web3 development workflow
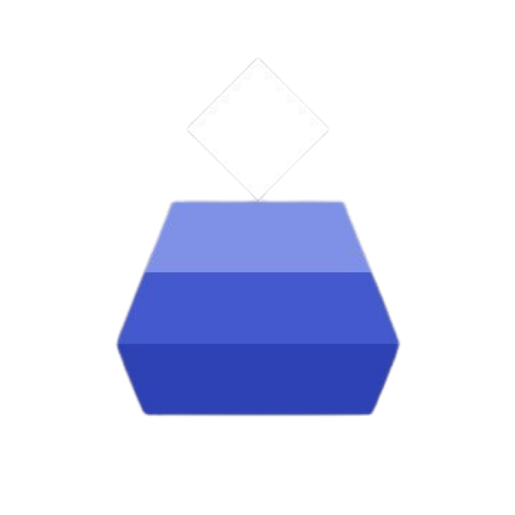
I'd be happy to help you create a simple MEV bot that monitors DEX trades for arbitrage opportunities. Here's a basic implementation:
const ethers = require('ethers');
require('dotenv').config();
// Set up provider and wallet
const provider = new ethers.providers.WebSocketProvider(
process.env.WSS_ENDPOINT
);
const wallet = new ethers.Wallet(process.env.PRIVATE_KEY, provider);
// DEX addresses and ABIs (simplified)
const dexes = [
{
name: 'Uniswap V2',
router: '0x7a250d5630B4cF539739dF2C5dAcb4c659F2488D',
factory: '0x5C69bEe701ef814a2B6a3EDD4B1652CB9cc5aA6f',
},
// Add other DEXes here
];
async function monitorArbitrageOpportunities() {
console.log('Starting MEV bot for arbitrage monitoring...');
// Listen for swap events from DEXes
const uniswapFactory = new ethers.Contract(
dexes[0].factory,
['event PairCreated(address indexed token0, address indexed token1, address pair, uint)'],
provider
);
// Listen for new trading pairs
uniswapFactory.on('PairCreated', async (token0, token1, pairAddress) => {
console.log(`New pair detected: ${token0} - ${token1}`);
monitorPair(pairAddress, token0, token1);
});
// Setup listeners for existing pairs
// Code to fetch and monitor existing pairs...
}
async function monitorPair(pairAddress, token0Address, token1Address) {
const pairAbi = [
'event Swap(address indexed sender, uint amount0In, uint amount1In, uint amount0Out, uint amount1Out, address indexed to)'
];
const pairContract = new ethers.Contract(pairAddress, pairAbi, provider);
pairContract.on('Swap', async (sender, amount0In, amount1In, amount0Out, amount1Out, to) => {
try {
// Check for arbitrage opportunity across different DEXes
const opportunity = await checkArbitrageOpportunity(
token0Address,
token1Address,
amount0In,
amount1In,
amount0Out,
amount1Out
);
if (opportunity.profitable) {
console.log('Arbitrage opportunity found!');
// Execute the arbitrage
await executeArbitrage(opportunity);
}
} catch (error) {
console.error('Error processing swap event:', error);
}
});
}
async function checkArbitrageOpportunity(token0, token1, amount0In, amount1In, amount0Out, amount1Out) {
// Compare prices across DEXes
// Calculate potential profit
// Return opportunity details if profitable
// Placeholder logic
return { profitable: false };
}
async function executeArbitrage(opportunity) {
// Bundle transactions
// Calculate gas costs
// Execute if profitable after gas
// Submit transaction
// Code to execute the arbitrage...
}
// Start monitoring
monitorArbitrageOpportunities().catch(console.error);
This is a simplified version to get you started. For a production-ready MEV bot, you would need to add:
- Gas optimization strategies
- Flash loan integration for higher capital efficiency
- Slippage protection
- More sophisticated pricing algorithms
Would you like me to elaborate on any specific part of this implementation?
Frequently Asked Questions
Get BaseUp updates directly to your inbox
Subscribe to receive email notifications whenever @_Base posts on X/Twitter